ESP8266 Sensor Bot
ESP8266, thingspeak.com, DHT11 sensor data interacting with SignalWire's AI technology
On this page, you'll find a full breakdown of the prompts used, along with all the functions. The functions are written in Perl. To quickly access all relevant files, please visit the GitHub repository.
It's dangerous to go alone!
This demo requires an ESP8266 SoC, and the DHT11 humidity and temperature sensor.
For a more straightforward demo that uses public APIs and has no hardware requirements, check out the Weather Bot demo.
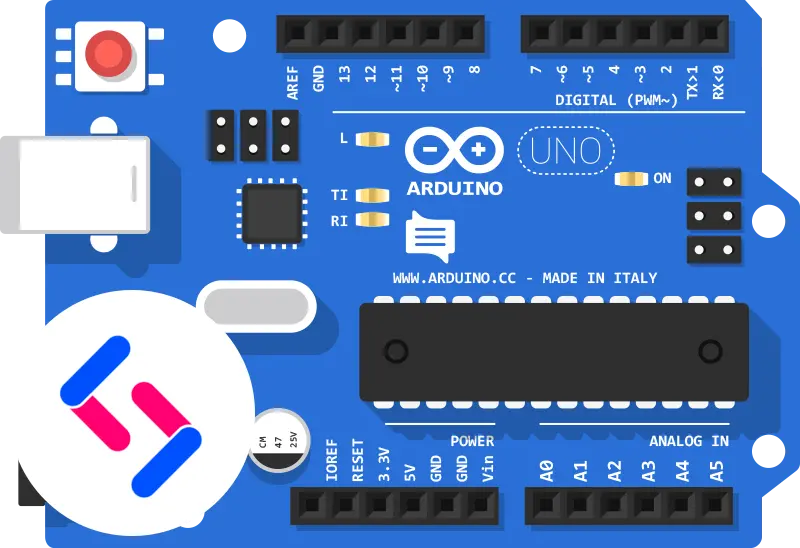
GitHub Repository
View the project and clone it to your development environment from the GitHub repository.
sensor_data Function
Perl modules:
LWP::Simple
for fetching data from the web,JSON
for parsing JSON data,Plack
for creating a simple web application, andSignalWire::ML
for constructing responses (assuming this is part of your application's architecture).
Step-by-Step Guide
1. Setting Up Your Perl Script
First, ensure you have Perl installed on your system along with the necessary modules. You can install missing modules using CPAN:
cpan install LWP::Simple JSON Plack
Start by importing the required modules at the beginning of your script:
use strict;
use warnings;
use Plack::Request;
use Plack::Response;
use SignalWire::ML;
use JSON qw(decode_json);
use LWP::Simple qw(get);
2. Fetching JSON Data
Define the URL of the JSON data you intend to fetch. In this example, we're using ThingSpeak's API to get the latest two entries of environmental data from a specific channel:
my $json_url = "https://api.thingspeak.com/channels/1464062/feeds.json?results=2";
Use LWP::Simple
's get
function to fetch the data:
my $json_text = get($json_url);
die "Could not fetch JSON data" unless defined $json_text;
3. Processing the JSON Data
Decode the fetched JSON text into a Perl data structure using the JSON
module:
my $decoded_json = decode_json($json_text);
Extract the necessary data from the decoded JSON. For instance, to get the temperature and humidity from the latest feed entry:
my $latest_feed = $decoded_json->{feeds}[-1];
my $temperature = $latest_feed->{field1};
my $humidity = $latest_feed->{field2};
4. Constructing the HTTP Response
Create a new response object with Plack::Response
and set the content type to application/json
:
my $res = Plack::Response->new(200);
$res->content_type('application/json');
Construct the response body to include the temperature and humidity information:
my $response_text = "The latest temperature is $temperature degrees, and the humidity is $humidity%.";
$res->body($swml->swaig_response_json({ response => $response_text }));
5. Finalizing the Response
Return the finalized response from your application:
return $res->finalize;
GitHub
Check out the full project repository for the Arduino Sensor Bot demo on GitHub.
SignalWire
SignalWire’s AI Agent for Voice allows you to build and deploy your own digital employee. Powered by advanced natural language processing (NLP) capabilities, your digital employee will understand caller intent, retain context, and generally behave in a way that feels “human-like”. In fact, you may find that it behaves exactly like your best employee, elevating the customer experience through efficient, intelligent, and personalized interactions.
Made with ❤️ by SignalWire's DevEx team
Have feedback on this site? Drop us a line at devex@signalwire.com or visit the Community Slack.